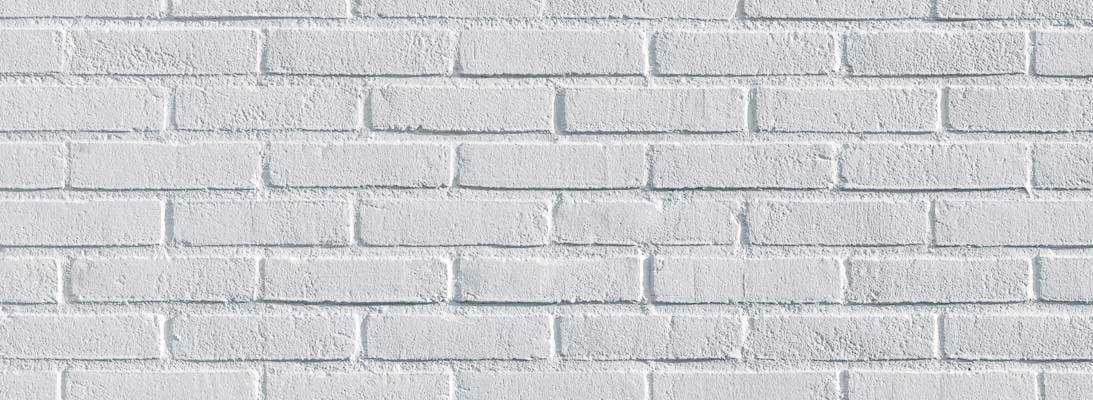
Array parameter in WebAPI Get Request
REST APIs are great, and ASP.NET Web API is a nice way of creating one. The auto-generated code that Visual Studio gives you will also get you 90% of the way there to knowing how to build one without reading much documentation.
However, while it will give you all the GET, POST, PUT, DELETE etc endpoints there's one thing it doesn't give you an example for, and that's query-string parameters on your GET request.
In the real world, the likelihood of you wanting the GET route to return everything is quite slim. You may have small datasets where this is true but more often than not, you're going to want some sort of query string values to use as a filter.
Fortunately, ASP.NET makes this simple with parameter binding. Just add them as parameters to your function.
1// GET: api/<People>2[HttpGet]3public IEnumerable<Person> Get(int? age, string? gender)4{5 ...6}
Make sure to make them nullable if you don't want them as required fields.
Swagger will even pick up on them and include them as parameter options.
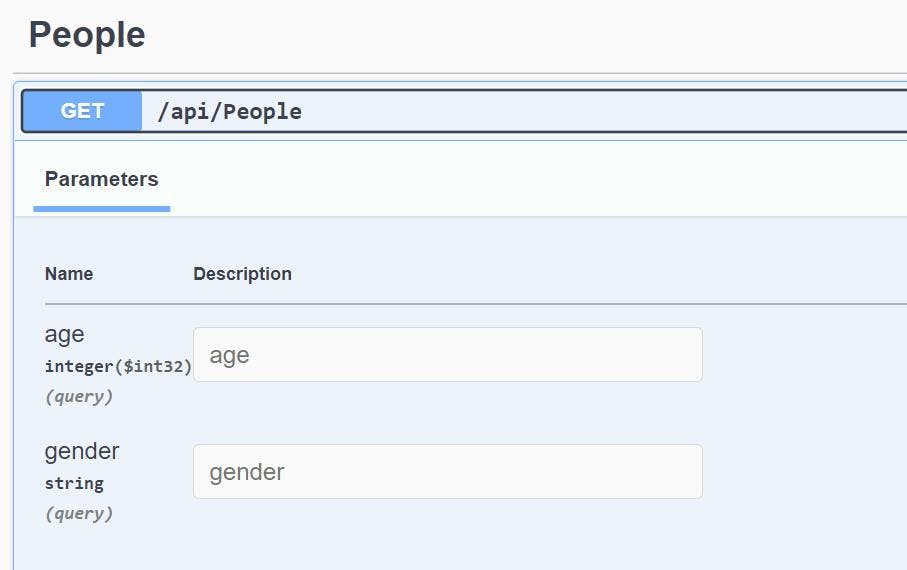
It's also likely that you'll want an array of values so that in my example you could select multiple ages or genders. However, if you just try turning them into arrays you will end up with this build error.
1'API.Controllers.PeopleController.Get (API)' has more than one parameter that was specified or inferred as bound from request body. Only one parameter per action may be bound from body. Inspect the following parameters, and use 'FromQueryAttribute' to specify bound from query, 'FromRouteAttribute' to specify bound from route, and 'FromBodyAttribute' for parameters to be bound from
If we only had one array things would be ok as the error is for specifying multiple. Except if we look at swagger things still aren't quite right.
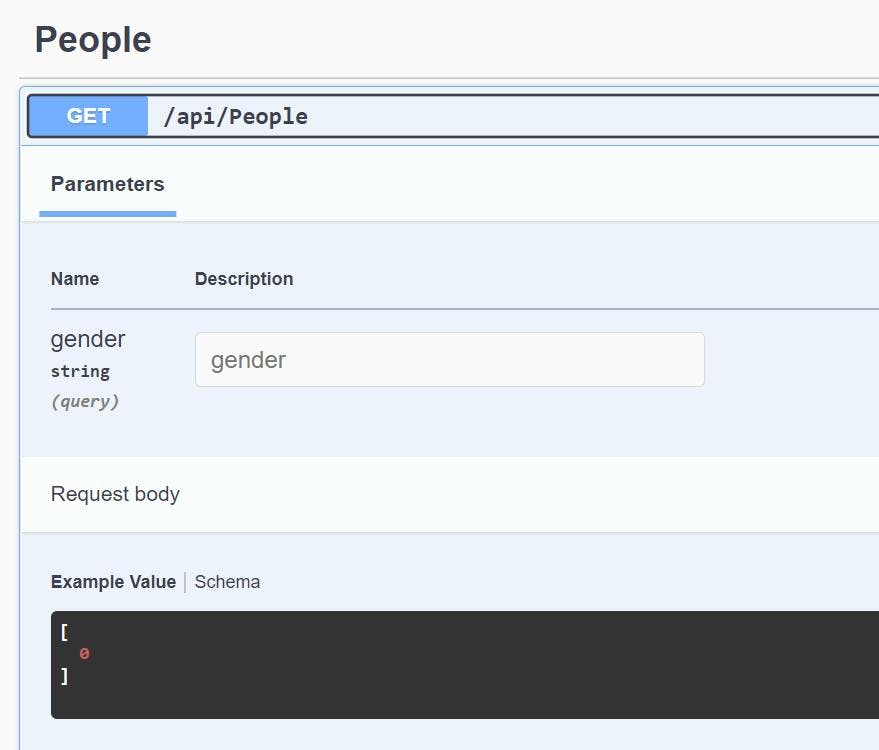
The age parameter is now showing as coming from the body rather than the query-string. It also explains why you could only specify one array as a parameter.
The solution is to specify that these parameters should come from the query-string which we can do by using the [FromQuery] attribute.
1// GET: api/<PeopleController>2[HttpGet]3public IEnumerable<Person> Get([FromQuery] int[]? age, [FromQuery] string[]? gender)4{5 ...6}
The solution will now run even with multiple array parameters, and swagger will also pick up that these are query-string array parameters and give you the option to add individual items when you test a request.
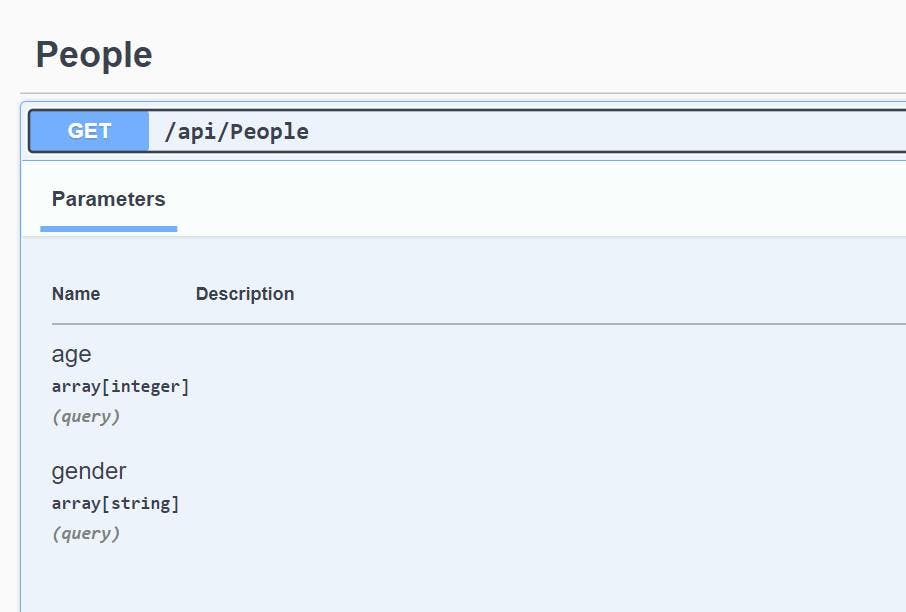