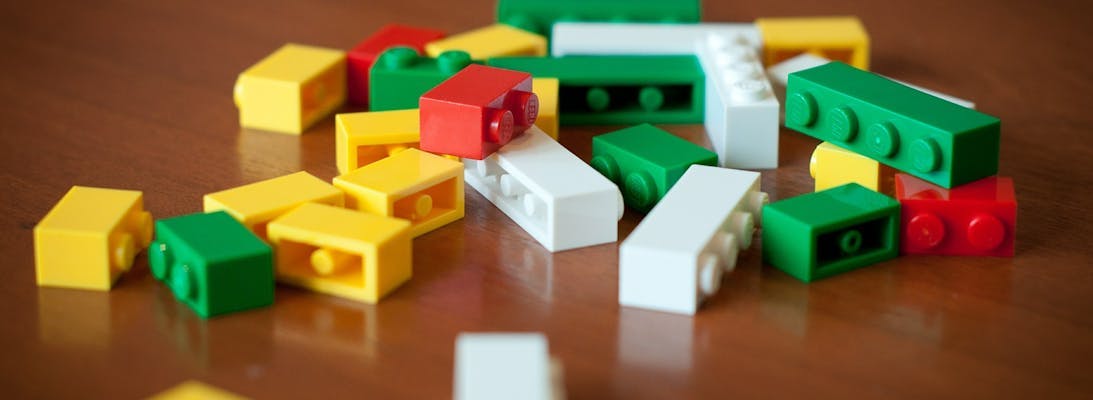
Creating a WFFM Save action with Field Mappings
The Sitecore Web Form for Marketers module offers content editors a flexible way to create data capture forms and then trigger certain actions to occur on submission. There's a whole host of save action options out the box, such as sending an email, enrolling the user in an engagement plan or updating some user details.
However one save action that is often required is the ability to send the data onto a CRM system so that it can get to the people that need to act on it, rather than staying in Sitecore with the content editors. To do this your best option is to create a custom save action that can send on the information.
Creating a Save Action in Sitecore
Save actions in Sitecore are configured under /sitecore/system/Modules/Web Forms for Marketers/Settings/Actions/Save Actions. Here you can see all the standard ones that come out the box and add your own.
Right click Save Actions and insert a new Save Action. You will need to fill out the Assembly and Class fields so that Sitecore knows which bit of code to execute (details on creating this bit below).
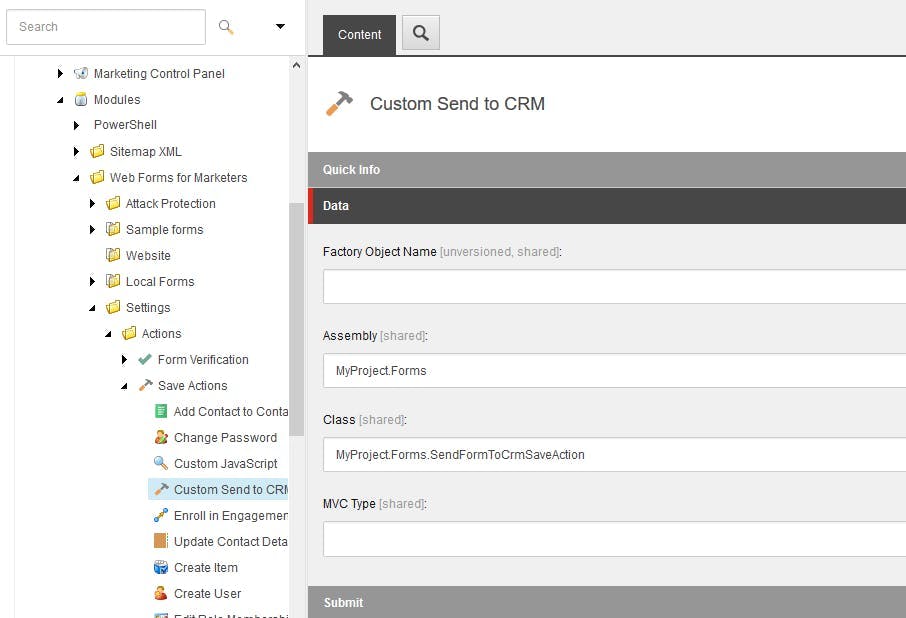
Adding Field Mappings
To really make your save action usable you will want to allow the content editor to map the fields on their form with the ones in the CRM, rather than relying on them both having the same name and hard coding the expected WFFM field names in your save action logic.
On your Save Action Item in Sitecore their are 2 fields to fill out to enable the editor in WFFM (Editor and QueryString). Luckily Sitecore provide a mapping editor out the box so there's very little effort involved here.
Within the Editor filed add the value:
1control:Forms.MappingFields
And within the querystring field, add your list of fields in the format fields=FieldName|FieldDisplayText
1fields=FirstName|First Name,LastName|Last Name,EmailAddress|Email Address,CompanyName|Company Name
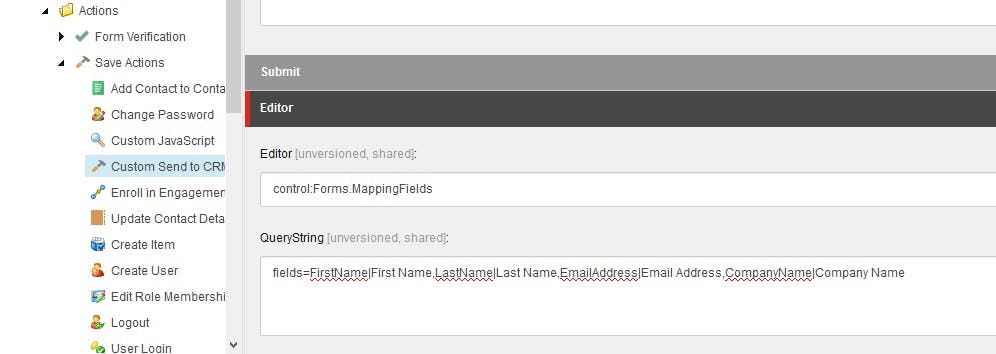
When the content editor now adds the save action to their form they will now be able to select a form field for each of these fields.
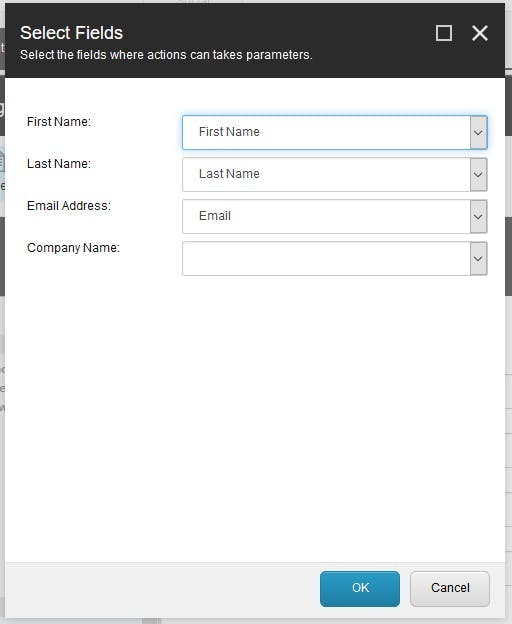
Creating the Save Action in code
To create the save action you will need a class that inherits from either ISaveAction or WffmSaveAction. I've used WffmSaveAction as it already has some of the interface implemented for you.
The field list you added to the Querystring property of the save action in Sitecore will need to be added as public properties to your class. Sitecore will then populate each of these with the ID the field gets mapped to or null if it has no mapping.
Then all that's left is to add an Execute method to populate your CRM's model with the data coming in through the adaptedFields parameter and send it onto your CRM.
1using Sitecore.Data;2using Sitecore.WFFM.Abstractions.Actions;3using Sitecore.WFFM.Actions.Base;4using System;56namespace MyProject.Forms7{8 public class SendFormToCrmSaveAction : WffmSaveAction9 {10 public string EmailAddress { get; set; }11 public string FirstName { get; set; }12 public string LastName { get; set; }13 public string CompanyName { get; set; }1415 public override void Execute(ID formId, AdaptedResultList adaptedFields, ActionCallContext actionCallContext = null, params object[] data)16 {17 // Map values from adapted fields into enquiry model18 IEnquiry enquiry = new Enquiry();1920 enquiry.Email = GetValue(this.EmailAddress, adaptedFields);21 enquiry.FirstName = GetValue(this.FirstName, adaptedFields);22 enquiry.LastName = GetValue(this.LastName, adaptedFields);23 enquiry.CompanyName = GetValue(this.CompanyName, adaptedFields);2425 // Add logic to send data for CRM here26 }2728 /// <summary>29 /// Get Value from field list data for a given form field id, or return null if not found30 /// </summary>3132 /// <param name="formFieldId"></param>33 /// <param name="fields"></param>34 /// <returns></returns>35 private string GetValue(string formFieldId, AdaptedResultList fields)36 {37 if (string.IsNullOrWhiteSpace(formFieldId))38 {39 return null;40 }41 if (fields == null || fields.GetEntryByID(formFieldId) == null)42 {43 return null;44 }45 return fields.GetValueByFieldID(formFieldId);46 }47 }48 }49}