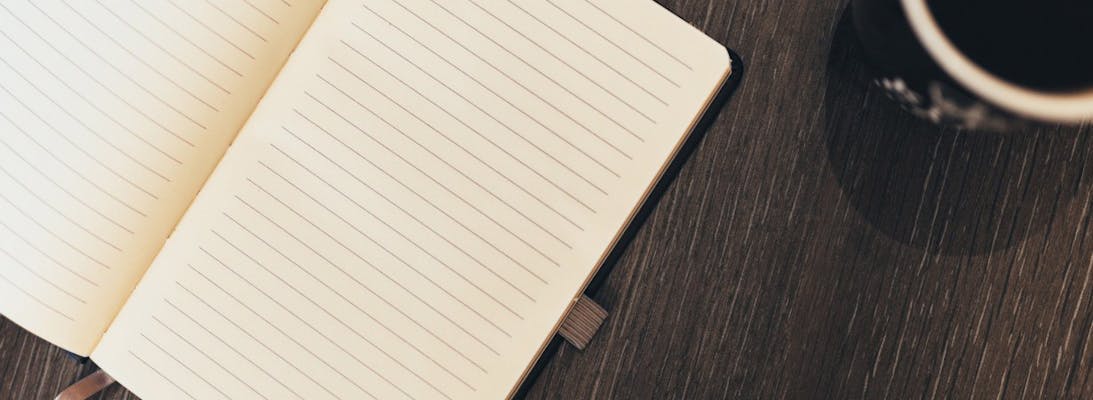
Pipelines - remember the big picture
Sitecore pipelines are great. With them you can relatively easily add and remove functionality as you wish. Pipelines like httpRequestBegin, httpRequestProcessed and mvc.beginRequest are also really useful if you need some logic to run on a page load that shouldn't really be part of a rendering. This could be anything from login checks to updating the way 404 pages are returned. However you do need to remember the big picture of what you are changing.
Pipelines don't just effect the processes of the website your building, Sitecore uses them too. That means when you add a new processor to the httpRequestBegin pipeline, that's going to effect every request in the admin CMS too. Just checking the context item also isn't enough as some things. e.g. opening a node in a tree view, will have the context of the node you clicked on!
Adding this snippet of code to the beginning of your process should keep you safe though.
1using Sitecore.Diagnostics;2using Sitecore.Pipelines.HttpRequest;3using Sitecore;4using Sitecore.SecurityModel;56namespace CustomPiplelineNamespace7{8 public class CustomPipeline : HttpRequestProcessor9 {10 public override void Process(HttpRequestArgs args)11 {12 //Check args isn't null13 Assert.ArgumentNotNull(args, "args");14 //Check we have a site15 if (Context.Site == null)16 return;17 //Check the sites domain isn't one for sitecore18 if (Context.Site.Domain != DomainManager.GetDomain("sitecore"))19 return;20 //Check that we're not in a redirect21 if (args.Url.FilePathWithQueryString.ToUpperInvariant().Contains("redirected=true".ToUpperInvariant()))22 return;23 //Check that we're not in the page editor24 if (Context.PageMode.IsPageEditor)25 return;2627 // DO CODE28 }29 }30}