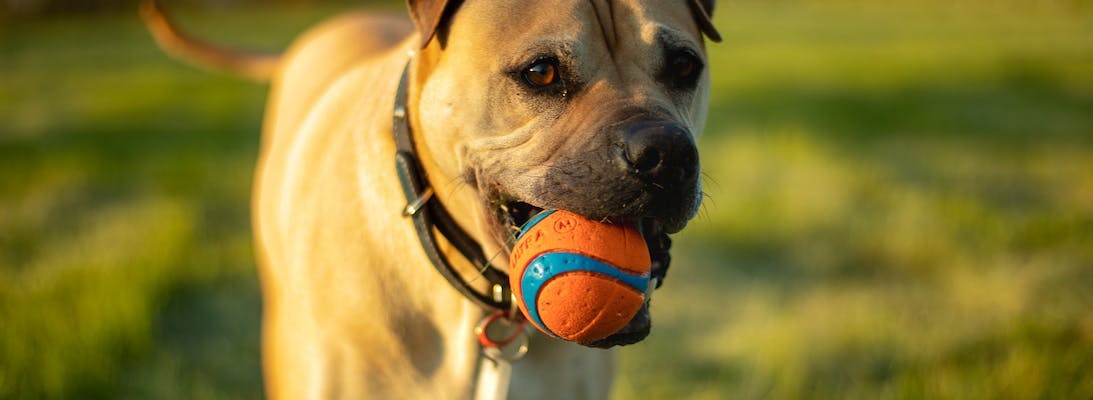
Data Fetching options with NextJS
When it comes to building websites in node, I'm a big fan of using NextJS. There's a lot of static site generators around but one of the things I really like about NextJS is not only the fact you have different options for data fetching, but that they're also really easy to use.
Static Generation
If you've somehow missed it, static site generation is the idea that to make your site run as fast as possible for every user, you pre-generate all the pages so that when a visitor makes a request for the page, all the server needs to do is return a file. It's a bit like the start of the internet when everything was an html file, before we became clever and started generating files dynamically on the fly. With all the processing removed, the time to first byte is greatly reduced.
To do this with NextJs you add a function called getStaticProps to your page and when the site is built, this will get all the static content and make it available to the page to be pre-generated.
1export async function getStaticProps(context) {2 return {3 props: {}, // will be passed to the page component as props4 }5}
Or if your using TypeScript.
1import { GetStaticProps } from 'next'23export const getStaticProps: GetStaticProps = async (context) => {4 // ...5}
Static Generation for Dynamic Paths
So that last example would generate content for a page, but what if you have a headless CMS and unlimited pages. You certainly couldn't create a file for each one with it's own getStaticProps function.
For this there is getStaticPaths. Like getStaticProps this function will run at the build time and is used to find and return all the dynamic paths for the page. Think of your page as the page template and this function is getting all the pages that relate to it. This is how I generate all the blog post pages on this site.
The function returns an object with two values. First paths which is a list of all the routes and the parameters for them. e.g. a list of the id's to get the data for a page with. The second is fallback: false, this tells NextJs that if the request is for a route not in the paths list, then returns a 404.
1export async function getStaticPaths() {2 return {3 paths: [4 { params: { ... } }5 ],6 fallback: false7 };8}
The params are then passed to the getStaticProps function so that it can pre-generate all the page content.
1// This also gets called at build time2export async function getStaticProps({ params }) {3 // params contains the post `id`.4 // If the route is like /posts/1, then params.id is 15 const res = await fetch(`https://.../posts/${params.id}`)6 const post = await res.json()78 // Pass post data to the page via props9 return { props: { post } }10}
If you'd prefer Typescript then this is the alternative.
1import { GetStaticPaths } from 'next'23export const getStaticPaths: GetStaticPaths = async () => {4 // ...5}
The Good Old Dynamic Way
Static's great, but sometimes are pages just aren't static enough for it to make sense. Here's where getServerSideProps comes in. With getServerSideProps your page will get generated on the server at runtime.
At this point you head may explode because at some point someone told you static site generators were faster, more secure etc because nothing runs on the server. Well erm.................. there is a server and it can generate files, so they were er... wrong.
1export async function getServerSideProps(context) {2 return {3 props: {}, // will be passed to the page component as props4 }5}
Or with TypeScript.
1import { GetServerSideProps } from 'next'23export const getServerSideProps: GetServerSideProps = async (context) => {4 // ...5}
Incremental Static Regeneration
Lets take are mind back to that first static page generation. It's great for the end user, but the downside is that each time you need to change something on a page you have to regenerate the entire site. On a big site, that's an issue.
Incremental Static Regeneration lets you tell NextJs that while you want the page the be pre-built, you also want that version to expire and get generated again. Think of this like the expiry time on a CDN, but configured at a page level in your site.
To use it the getStaticProps function needs to return a revalidate value. This gives the instruction of up to how frequently the page should be generated.
1export async function getStaticProps() {2 const res = await fetch('https://.../posts')3 const posts = await res.json()45 return {6 props: {7 posts,8 },9 // Next.js will attempt to re-generate the page:10 // - When a request comes in11 // - At most once every 10 seconds12 revalidate: 10, // In seconds13 }14}
Part Static Generation
So lets say we don't need our pages to expire other than when we rebuild the site, and we also don't want to generate every page in our site because there 2000 of them and it will take ages, but there's also some really important pages that we don't want any user to have to wait for.
Well if we go back to our static generation for dynamic routes example and take another look at that fallback value we can do something different.
Fallback can be set to 3 values, false, true and blocking. False will return a 404 if the route didn't exist, but true and blocking gives us an option to decide at runtime if we want to generate a page or issue a 404.
Setting fallback to true will return a fallback page, getStaticProps will then generate the page and this will then get swapped with the fallback version. Blocking is similar but will wait for the new page to be generated rather than initially issuing a fallback.
By doing this, the paths we return in the props will get generated at build time (we can restrict this to just our important pages), and all the others will function like a regular CDN.
Summary
So as you can see, there's a whole host of options to fetching data, when you do it, how you do it, how long it lives for and I didn't even cover the fact you could still fetch data dynamically when the page has loaded on the client! If we did this then we can pre-generate all the static parts of our page and then populate other bits dynamically after on the client.