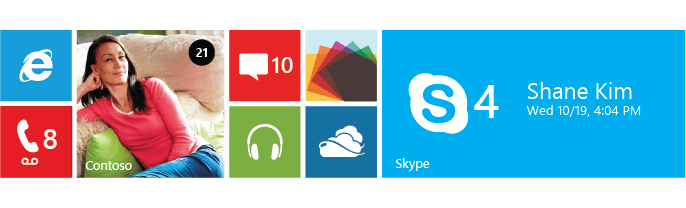
Creating Training Buddy's Live Tile
Live tiles are probably the best known feature about Windows Phone. Unlike iOS's plain grid of app icons Microsoft designed their phones home screen to provide users with data without having to open the app. With Windows Phone 8 the feature was updated so that 3rd party apps like Training Buddy could offer full width tiles along with new layout templates.
Adding a live tile to your app is a great idea as it is one of the features users often look for when their choosing an app. The app store also handily points out if an app uses a live tile or not.
The Design
There are 3 tile templates to choose from when you add a live tile to your app. These are Flip, Iconic and Cycle.
Flip gives the illusion that the tile has a front and a back and will flip over after a few seconds.
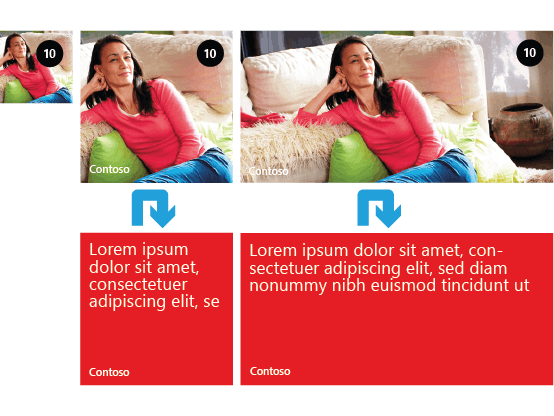
Iconic has space for an image and a large number, a bit like the icon for messages and emails. When in its largest size there is also wide content zones that can contain text.
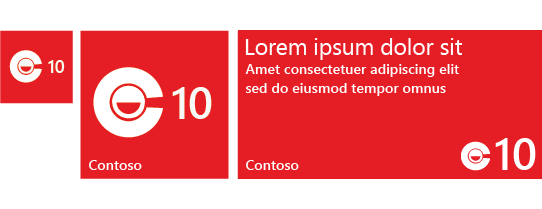
Cycle lets you choose 9 images that the app will Cycle through.
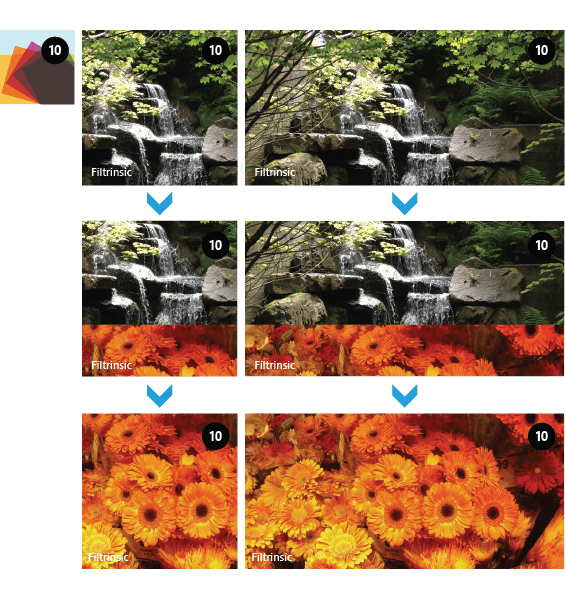
For Training Buddy I have used the Iconic template. You will probably find like myself that the type of app you are creating will more than likely determine what template you are going to use. As Training Buddy's live tile was ultimately going to show details of the users last activity, Iconic was the obvious choice. The smaller sizes don't really have enough space to give any activity stats and the large version gives you an additional space for a smaller image that was perfect for the activity type image (running, cycling, walking).
Another alternative is to make a completely custom design and write something in your app to render it as an image. You can then display the image using either the flip or cycle template.
The Code
The second reason you everyone should add live tiles to their app is because the code is so simple (this is the actual code from Training Buddy).
1// Application Tile is always the first Tile, even if it is not pinned to Start.2 ShellTile TileToFind = ShellTile.ActiveTiles.First();34 // Application should always be found5 if (TileToFind != null)6 {7 string WideContent1 = "";8 string WideContent2 = "";9 string WideContent3 = "";10 string activityLogo = "";11 if (App.settings.LiveTile)12 {13 var lastActivity = (from a in AllActivities14 orderby a.StartDateTime descending15 select a).Take(1);1617 if (lastActivity.Count() > 0)18 {19 if (App.settings.DistanceMeasurement == "Miles")20 {21 WideContent3 = "Distance: " + lastActivity.First().Distance.ToString("0.##") + " miles";22 }23 else24 {25 WideContent3 = "Distance: " + (lastActivity.First().Distance * 1.609344).ToString("0.##") + " km";26 }27 WideContent2 = "Date: " + lastActivity.First().StartDateTime.ToShortDateString();28 switch (lastActivity.First().ActivityType.ToLower())29 {30 case "running":31 WideContent1 = "Last Run";32 break;33 case "walking":34 WideContent1 = "Last Walk";35 break;36 case "cycling":37 WideContent1 = "Last Cycle";38 break;39 case "swimming":40 WideContent1 = "Last Swim";41 break;42 }4344 activityLogo = "/Assets/" + lastActivity.First().ActivityType + "Black-70.png";4546 if (lastActivity.First().CaloriesBurned > 0)47 {48 WideContent3 += " Calories: " + lastActivity.First().CaloriesBurned.ToString("0.#");49 }5051 }5253 }5455 IconicTileData tileDate = new IconicTileData56 {57 Title = "Training Buddy",58 WideContent1 = WideContent1,59 WideContent2 = WideContent2,60 WideContent3 = WideContent3,61 IconImage = new Uri("/Assets/RunningBlack-150.png", UriKind.Relative),62 SmallIconImage = new Uri(activityLogo, UriKind.Relative)63 };6465 // Update the Application Tile66 TileToFind.Update(tileDate);67 }
First I'm finding the application tile. It is possible to create additional tiles for your app which is another great feature, but if you want to just update the main tile it will be the first one returned.
Next I'm checking to see if the user has turned on the live tile or not. If they haven't then I'm just setting the tile back to its default state.
The following lines are then getting the content to display on the tile and building up the strings on local variables.
Lastly and most importantly I'm creating a new instance of IconicTileData and setting each of its properties with the data to show. Then it's just a case of calling Update on the tile instance and providing it with the new IconicTileData object.
The Tile
And here's the result
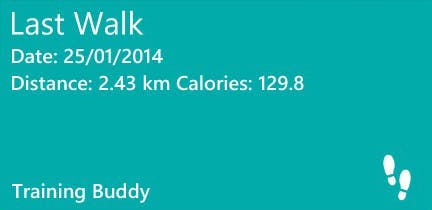
Live tiles are really easy to create so if your developing an app you should definitely take the time to add one.