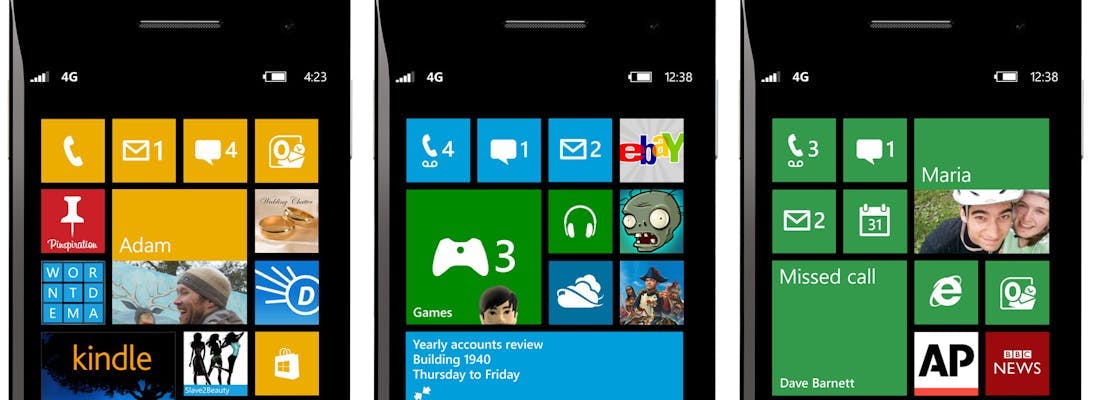
Theme Manager for Windows Phone
One of Windows Phones features is the ability for a user to switch between light and dark themes. However as a developer creating a good experience in your apps that looks good in both themes can be a challenge. What's worse is it sometimes leads to apps that look great in one theme but not the other.
Unfortunately there is also no official easy way to tell the phone you want your app to run with a particular theme. Instead it's a manual process of either setting the colour of every object, or manually loading a theme in the apps start-up.
Thankfully there is a better way and it comes in the form of a NuGet package. By simply installing the wp-thememanager package you can call ThemeManager.ToLightTheme() in your apps contructor to make the theme light, or ThemeManager.ToDarkTheme() to make it dark.
1/// <summary>2/// Constructor for the Application object.3/// </summary>4public App()5{6 // Global handler for uncaught exceptions.7 UnhandledException += Application_UnhandledException;89 // Standard Silverlight initialization10 InitializeComponent();1112 // Phone-specific initialization13 InitializePhoneApplication();1415 ThemeManager.ToLightTheme();1617 // Other code that might be here already...18}