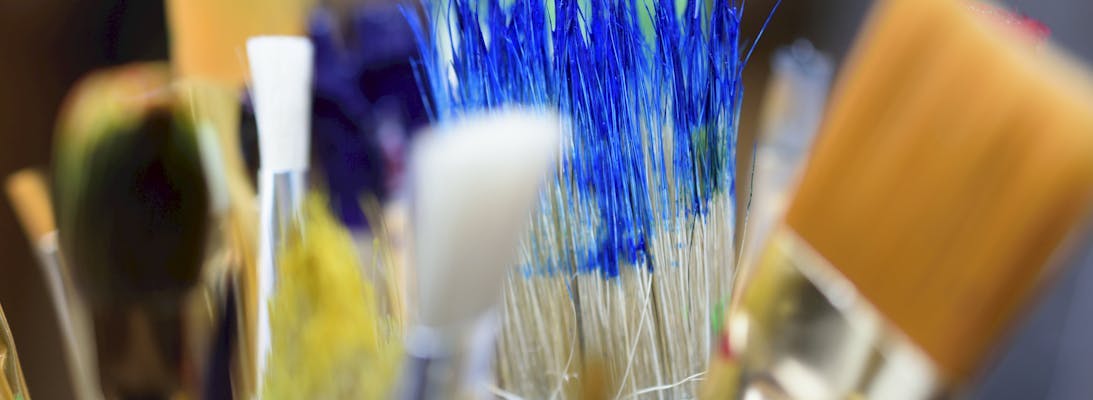
Force clients to refresh JS/CSS files
It's a common problem with an easy solution. You make some changes to a JavaScript of CSS file, but your users still report an issue due to the old version being cached.
You could wait for the browsers cache to expire, but that isn't a great solution. Worse if they have the old version of one file and the new version of another, there could be compatibility issues.
The solution is simple, just add a querystring value so that it looks like a different path and the browser downloads the new version.
Manually updating that path is a bit annoying though so we use modified time from the actual file to add the number of ticks to the querystring.
UrlHelperExtensions.cs
1using Utilities;2using UrlHelper = System.Web.Mvc.UrlHelper;34namespace Web.Mvc.Utils5{6 public static class UrlHelperExtensions7 {8 public static string FingerprintedContent(this UrlHelper helper, string contentPath)9 {10 return FileUtils.Fingerprint(helper.Content(contentPath));11 }12 }13}
FileUtils.cs
1using System;2using System.IO;3using System.Web;4using System.Web.Caching;5using System.Web.Hosting;67namespace Utilities8{9 public class FileUtils10 {11 public static string Fingerprint(string contentPath)12 {13 if (HttpRuntime.Cache[contentPath] == null)14 {15 string filePath = HostingEnvironment.MapPath(contentPath);1617 DateTime date = File.GetLastWriteTime(filePath);1819 string result = (contentPath += "?v=" + date.Ticks).TrimEnd('0');20 HttpRuntime.Cache.Insert(contentPath, result, new CacheDependency(filePath));21 }2223 return HttpRuntime.Cache[contentPath] as string;24 }25 }26}