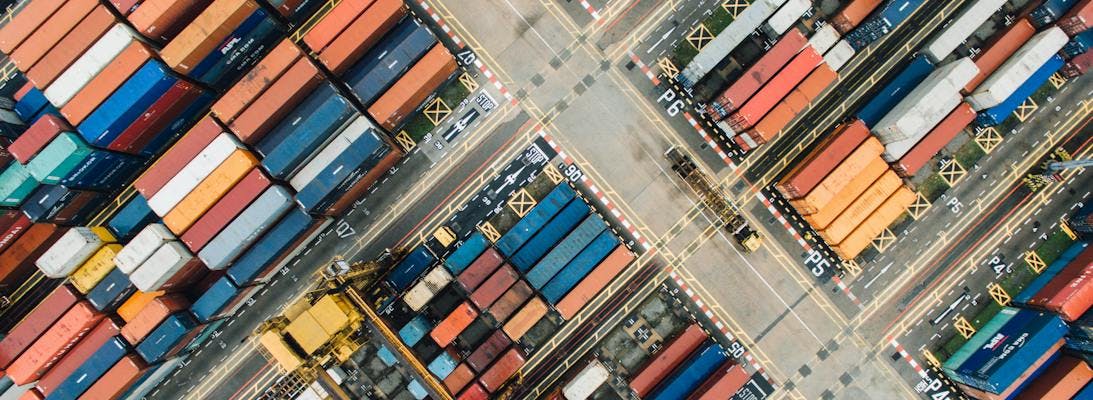
SonarQube for .NET Framework with GitHub Actions
If you haven't tried SonarQube or SonarCloud out then I suggest you do. The cloud version is quite straightforward to setup and from my experience the stuff it finds can be quite insightful. Like all these tools, at times you'll disagree with what they say, but there's always the option to change the rules.
What I particularly like with SonarQube is the examples you get with each bug that clearly explains why there's an issue and what you need to do in order to fix it.
What I didn't like however were the instructions for setting a project using .NET Framework. There are instructions labelled .NET, but this heavily assumes your using .NET Core, which while that might be our general preference, products like Sitecore could force your hand back to .NET Framework and all those legacy projects didn't just go away.
How to setup SonarQube using GitHub Actions for .NET Framework
The GitHub setup instructions (https://docs.sonarqube.org/latest/analysis/github-integration/) will give you the following code to create your GitHub Action with. This is also the same code you will get if you follow the wizard in SonarQube.
1name: Build2on:3 push:4 branches:5 - master # or the name of your main branch6 pull_request:7 types: [opened, synchronize, reopened]8jobs:9 build:10 name: Build11 runs-on: windows-latest12 steps:13 - name: Set up JDK 1114 uses: actions/setup-java@v115 with:16 java-version: 1.1117 - uses: actions/checkout@v218 with:19 fetch-depth: 0 # Shallow clones should be disabled for a better relevancy of analysis20 - name: Cache SonarQube packages21 uses: actions/cache@v122 with:23 path: ~\sonar\cache24 key: ${{ runner.os }}-sonar25 restore-keys: ${{ runner.os }}-sonar26 - name: Cache SonarQube scanner27 id: cache-sonar-scanner28 uses: actions/cache@v129 with:30 path: .\.sonar\scanner31 key: ${{ runner.os }}-sonar-scanner32 restore-keys: ${{ runner.os }}-sonar-scanner33 - name: Install SonarQube scanner34 if: steps.cache-sonar-scanner.outputs.cache-hit != 'true'35 shell: powershell36 run: |37 New-Item -Path .\.sonar\scanner -ItemType Directory38 dotnet tool update dotnet-sonarscanner --tool-path .\.sonar\scanner39 - name: Build and analyze40 env:41 GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} # Needed to get PR information, if any42 shell: powershell43 run: |44 .\.sonar\scanner\dotnet-sonarscanner begin /k:"example" /d:sonar.login="${{ secrets.SONAR_TOKEN }}" /d:sonar.host.url="${{ secrets.SONAR_HOST_URL }}"45 dotnet build46 .\.sonar\scanner\dotnet-sonarscanner end /d:sonar.login="${{ secrets.SONAR_TOKEN }}"
There's two aspects to notice with this. Firstly the Build and analyze section is running a command dotnet build which is fine if your running .Net Core, but for .Net Framework it isn't going to work.
Secondly it's highly likely your solution will use NuGet packages and there's no step in here to restore them.
To setup and restore NuGet packages add in the following steps before the Build and analyze step. Be sure to put your solution filename in the restore command.
1 - name: Setup Nuget2 uses: Nuget/setup-nuget@v1.0.534 - name: Restore nuget packages5 run: nuget restore MySolution.sln
To do a build that will compile your .Net Framework code you will need to use MsBuild rather than dotnet. However if you just swap them over you'll get an invalid command error. First you need to add msbuild to PATH. Change your build steps as follows.
1 - name: Add msbuild to PATH2 uses: microsoft/setup-msbuild@v1.0.234 - name: Build and analyze5 env:6 GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} # Needed to get PR information, if any7 shell: powershell8 run: |9 .\.sonar\scanner\dotnet-sonarscanner begin /k:"example" /d:sonar.login="${{ secrets.SONAR_TOKEN }}" /d:sonar.host.url="${{ secrets.SONAR_HOST_URL }}"10 dotnet build11 .\.sonar\scanner\dotnet-sonarscanner end /d:sonar.login="${{ secrets.SONAR_TOKEN }}"
With that now in place you can now compile some .Net Framework code and have the results sent back to your SonarQube instance.