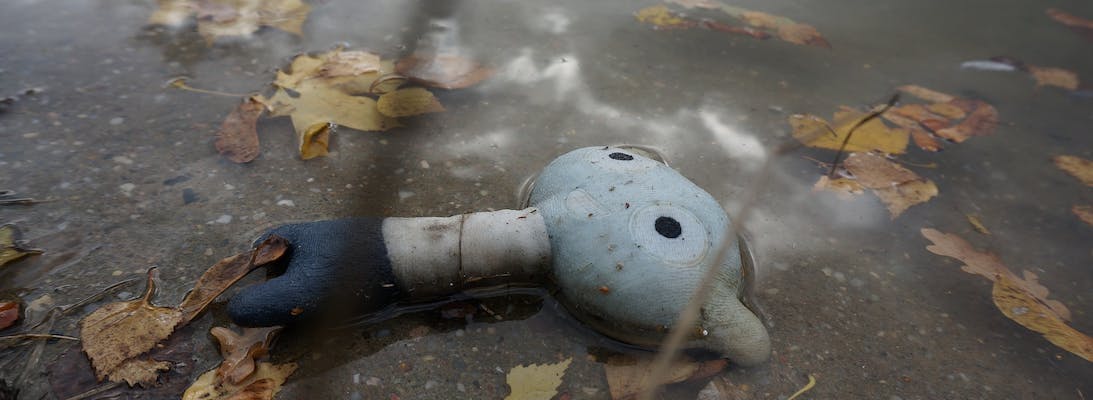
Sitecore: Returning a 404 response on the page requested rather than redirecting to a 404 page
Previously I've blogged about:
but while looking through the posts today, I realised I had never written about how you stop Sitecore from issuing 302 redirects to your 404 page and instead return a 404 on the URL requested with the contents of the 404 page.
While search engines will recognise a 302 response to a 404 as a 404 (in fact they're intelligent enough to work out that a 404 page without a correct response status code is a 404) it's considered SEO best practice for the URL to stay the same and to issue the correct status code.
Creating a NotFoundResolver class
When Sitecore processes a request it will run the httpRequestBegin pipeline, and within that pipeline is a Item Resolver processor that will attempt to find the requested item. If after this the context item is still null then the logic to redirect to the ItemNotFoundUrl will kick in. To stop this happening we can simply add our own process to the pipeline after ItemResolver and set the item.
Our class looks like this:
1using Sitecore;2using Sitecore.Configuration;3using Sitecore.Data;4using Sitecore.Diagnostics;5using Sitecore.Pipelines.HttpRequest;67namespace Pipelines.HttpRequest8{9 public class NotFoundResolver : HttpRequestProcessor10 {11 private static readonly string PageNotFoundID = Settings.GetSetting("PageNotFound");1213 public override void Process(HttpRequestArgs args)14 {15 Assert.ArgumentNotNull(args, nameof(args));1617 if ((Context.Item != null) || (Context.Database == null))18 return;1920 if (args.Url.FilePath.StartsWith("/~/"))21 return;2223 var notFoundPage = Context.Database.GetItem(new ID(PageNotFoundID));24 if (notFoundPage == null)25 return;2627 args.ProcessorItem = notFoundPage;28 Context.Item = notFoundPage;29 }30 }31}
To add our process to the pipeline we can use a patch file like this:
1<configuration xmlns:patch="http://www.sitecore.net/xmlconfig/">2 <sitecore>3 <pipelines>4 <httpRequestBegin>5 <processor6 patch:after="processor[@type='Sitecore.Pipelines.HttpRequest.ItemResolver, Sitecore.Kernel']"7 type="LabSitecore.Core.Pipelines.NotFoundResolver, LabSitecore.Core" />8 </httpRequestBegin>9 </pipelines>10 <settings>11 <!-- Page Not Found Item Id -->12 <setting name="PageNotFound" value="ID OF YOUR 404 PAGE" />13 </settings>14 </sitecore>15</configuration>
Notice the setting for the ID of your 404 page to be loaded as the content.
Remember if you do do this make sure you also follow one of the methods to return a 404 status code, otherwise you will have just made every URL a valid 200 response on your site.