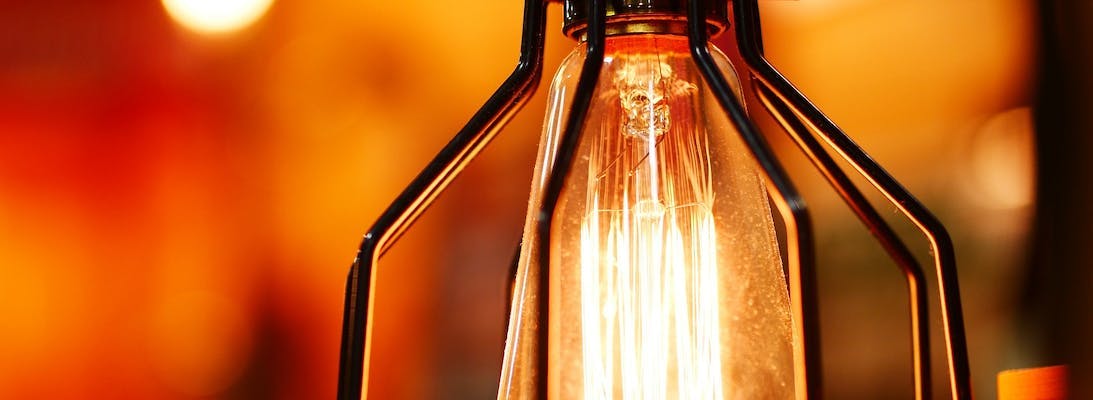
Checking Auth0 Roles and Permissions with a React SPA
With Auth0 you can set up a completely SAAS-based Authentication and Authorization provider, freeing you of any worries surrounding losing passwords, implementing the latest authentication best practices and a whole heap of other things.
One thing that tripped me up with it, however, was checking what permissions have been assigned to my users.
In my setup, I was using a .NET Core Web API hosted in Azure and a React SPA hosted separately. My API would need to check the permissions assigned to my users in order to check they could perform actions such as updating another user, and my React front end would need to show and hide various pieces of functionality to match.
Checking Permissions in the .NET Core Web API
This article isn't about permissions in APIs but it's important to understand how this differs too what's needed in the React front end.
The tutorial ASP.NET Core Web API v2.1: authoization is relatively straightforward to follow and at the end, you will have an API that performs Authorization with a user's permissions.
Permissions in Auth0 are assigned to users via roles. i.e. A user gets assigned a role and roles have related permissions. This way we can design our application around granular permissions and the roles which give those permissions to a user can be configured separately.
The permissions are provided to the API within the user's authentication token, however for this to work they actually have to be in the token to start with. By default roles and permissions are not included in a token.
These can be included by setting the scope property when calling getAccessTokenSilently (the function to get a token) in React.
1const token = await getAccessTokenSilently({2 authorizationParams: {3 audience: 'https://api.example.com/',4 scope: 'read:posts',5 },6});7const response = await fetch('https://api.example.com/posts', {8 headers: {9 Authorization: `Bearer ${token}`,10 },11});
Alternatively, you can Enable Role-Based Access Control for APIs. This will increase the size of your token by including all permissions assigned to the person, but removes the need to request them.
Checking Permissions in React
It's likely that before calling an API you will want your React logic to check the users permissions. After all, it wouldn't be a great user experience if they're allowed to perform actions which result in permission errors.
As well as the getAccessTokenSilently the useAuth0 React Hook contains a property for the user and getIdTokenClaims. When using these you will be able to see the details associated with the logged in user. e.g. Name. However unlike getAccessTokenSilently, it doesn't matter what you include in the scope or if you've enabled role-based access control, the properties will not include what permissions or roles are assigned to the user.
To include them you must setup a login action to add them to the token. See Add user roles to ID and Access tokens in Auth0's documentation.
This will direct you to create a login flow where you add a custom action containing the following.
1/**2 * @param {Event} event - Details about the user and the context in which they are logging in.3 * @param {PostLoginAPI} api - Interface whose methods can be used to change the behavior of the login.4 */5exports.onExecutePostLogin = async (event, api) => {6 const namespace = 'https://my-app.example.com';7 if (event.authorization) {8 api.idToken.setCustomClaim(`${namespace}/roles`, event.authorization.roles);9 api.accessToken.setCustomClaim(`${namespace}/roles`, event.authorization.roles);10 }11}
Note, the namespace part is so that what you add to the token is unique and doesn't clash with anything Auth0 is adding.
You can now follow these examples to perform a claims checks on the user. E.g.
1import { User } from '@auth0/auth0-react';23function checkClaims(claim?: User, role?: string) {4 return claim?.['https://my-app.example.com/roles']?.includes(role);5}67export default checkClaims;
This as you may have spotted is only assigning a role to the token rather than actual permissions. In my case, this was enough, however, this is one area I think the react hook is severely lacking.
Writing custom logic to set a users permissions into the set of claims feels highly wrong, but after some back and forth with Auth0's support team, for now though this appears to be the only option.