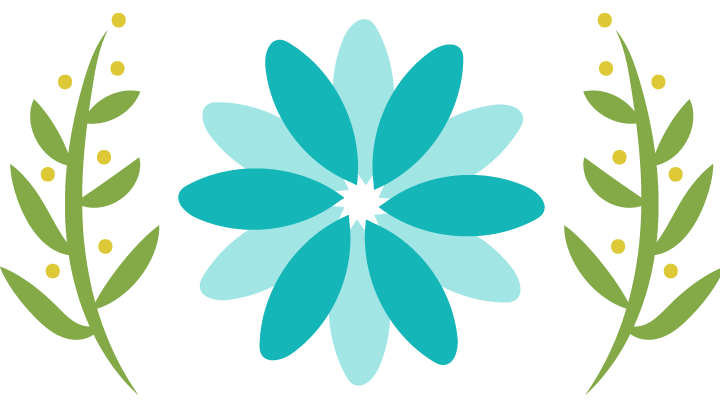
Displaying the cost of a uCommerce custom IShippingMethodService
I just found this bog post on how to get the shipping cost for a custom shipping method you may have implemented using IShippingMethodService and thought I would share it here.
http://www.davejsaunders.com/2014/10/20/display-the-cost-of-your-ucommerce-custom-shipping-service.html
A common requirement when displaying shipping methods is to show the price against each, and for the built in uCommerce shipping methods there's a handy GetPriceForCurrency() method on the shipping method object. However if that shipping method is a custom one, it just returns 0.
1var allShippingMethods = TransactionLibrary.GetShippingMethods(country);2var currency = purchaseOrder.BillingCurrency;34foreach (var shippingMethod in allShippingMethods)5{6 var cost = shippingMethod.GetPriceForCurrency(currency);7}
The correct way to get your shipping price is to manually call your service to get the price to be calculated.
As we don't know which implementation of IShippingMethodService will be used we first need to call GetShippingMethodService() to get it.
After that we just need to populate a new Shipment object we the details our custom method needs and then call the CalculateShippingPrice method.
1var purchaseOrder = TransactionLibrary.GetBasket().PurchaseOrder;2var allShippingMethods = TransactionLibrary.GetShippingMethods(country);34foreach (var shippingMethod in allShippingMethods)5{6 // Get the IShippingMethodService for this ShippingMethod7 var shippingService = shippingMethod.GetShippingMethodService();89 // Construct a fake shipping method to call the service with10 var shipment = new Shipment11 {12 ShippingMethod = shippingMethod,13 OrderLines = purchaseOrder.OrderLines,14 PurchaseOrder = purchaseOrder,15 ShipmentAddress = purchaseOrder.BillingAddress16 };1718 var shippingMethodPrice19 = shippingService.CalculateShippingPrice(shipment);20}