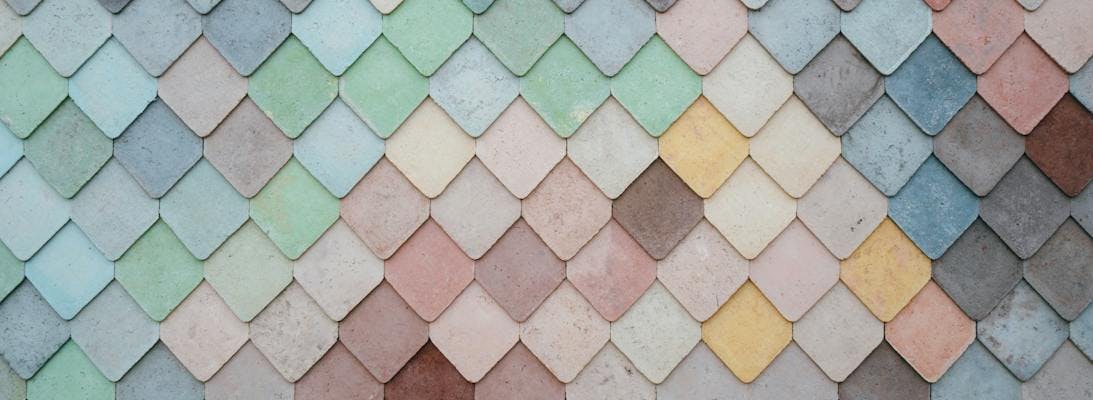
Debugging VueJS + TypeScript with VS Code - Part 2
In the past I have written about how to setup VS Code to debug a VueJS + TypeScript project. Since writing that article it's a method I've continued to use and it works well. It's quick to spin up and quite reliably allows you to place breakpoints in code.
However one aspect of it that I don't like is it's not so much "Run and Debug" from VSCode, it's more just the debug part, as to use it you must first go to a terminal and run your VueJS application.
There's two problems with this:
1. Most of the time you will just run the application not debugging (because why debug when you don't have an issue), therefore when you need it, you have to redo what you just did that caused an error. Instinctively there is then a desire to guess at what was wrong rather than going to the extra effort of debugging (frequently when you do this your guess is wrong and you end up spending more time guessing at what was wrong than using the tool that will tell you).
2. As the debugger generally isn't running, exceptions never get flagged up, and unless you have the browser console open (and check it), you can remain oblivious to something going wrong in your app.
There is a solution though, and it's quite simple!
Using VS Code to launch via npm
First follow through my previous guide on debugging a VueJS and TypeScript application.
Next, in your launch.config file add a new configuration with the definition below. This will run the command in a debug terminal, effectively doing the same thing as you typing npm run serve.
1{2 "command": "npm run serve",3 "name": "Run npm serve",4 "request": "launch",5 "type": "node-terminal"6 },
To get both our new and old configuration to run you can add a compound definition, that does both at the same time.
Here's mine.
1"compounds": [2 {3 "name": "Run and Debug",4 "configurations": ["Run npm serve", "vuejs: edge"]5 }6 ],
My complete file now looks like this. Note you don't need configurations for edge and chrome, just use the one for the browser you use.
1{2 // Use IntelliSense to learn about possible attributes.3 // Hover to view descriptions of existing attributes.4 // For more information, visit: https://go.microsoft.com/fwlink/?linkid=8303875 "version": "0.2.0",6 "compounds": [7 {8 "name": "Run and Debug",9 "configurations": ["Run npm serve", "vuejs: edge"]10 }11 ],12 "configurations": [13 {14 "command": "npm run serve",15 "name": "Run npm serve",16 "request": "launch",17 "type": "node-terminal"18 },19 {20 "type": "pwa-msedge",21 "request": "launch",22 "name": "vuejs: edge",23 "url": "http://localhost:8080",24 "webRoot": "${workspaceFolder}",25 "breakOnLoad": true,26 "sourceMapPathOverrides": {27 "webpack:///./*": "${webRoot}/*"28 },29 "skipFiles": ["${workspaceFolder}/node_modules/**/*"]30 },31 {32 "type": "chrome",33 "request": "launch",34 "name": "vuejs: chrome",35 "url": "http://localhost:8080",36 "webRoot": "${workspaceFolder}",37 "breakOnLoad": true,38 "sourceMapPathOverrides": {39 "webpack:///./*": "${webRoot}/*"40 },41 "skipFiles": ["${workspaceFolder}/node_modules/**/*"]42 }43 ]44}45
Now whenever you want to run the application, just run the compound Run and Debug and your VueJS app will start up and launch in your browser.