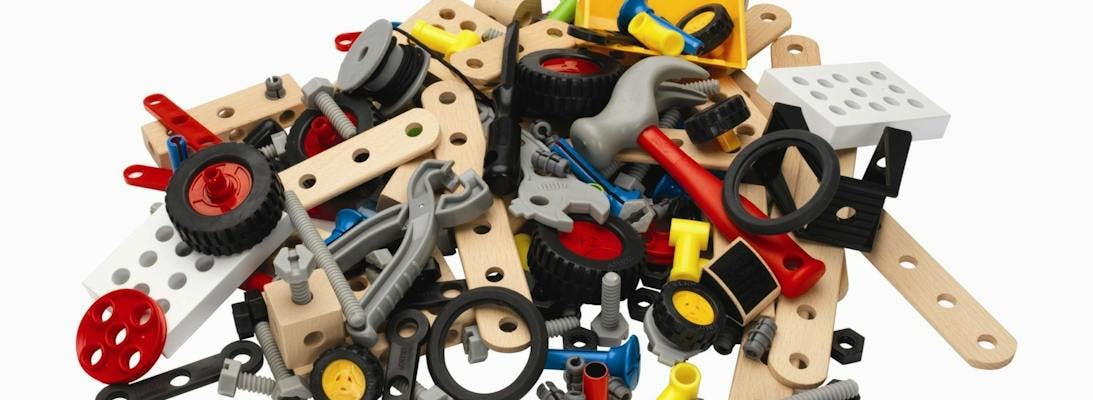
Populating the internal search report in Sitecore
Out the box Sitecore ships with a number of reports pre-configured. Some of these will show data without you doing anything. e.g. The pages report will automatically start showing the top entry and exit pages as a page view is something Sitecore can track.
Other's like the internal search report will just show a message of no data to display, which can be confusing/frustrating for your users. Particularly when they've just spent money on a license fee to get great analytics data only to see a blank report.
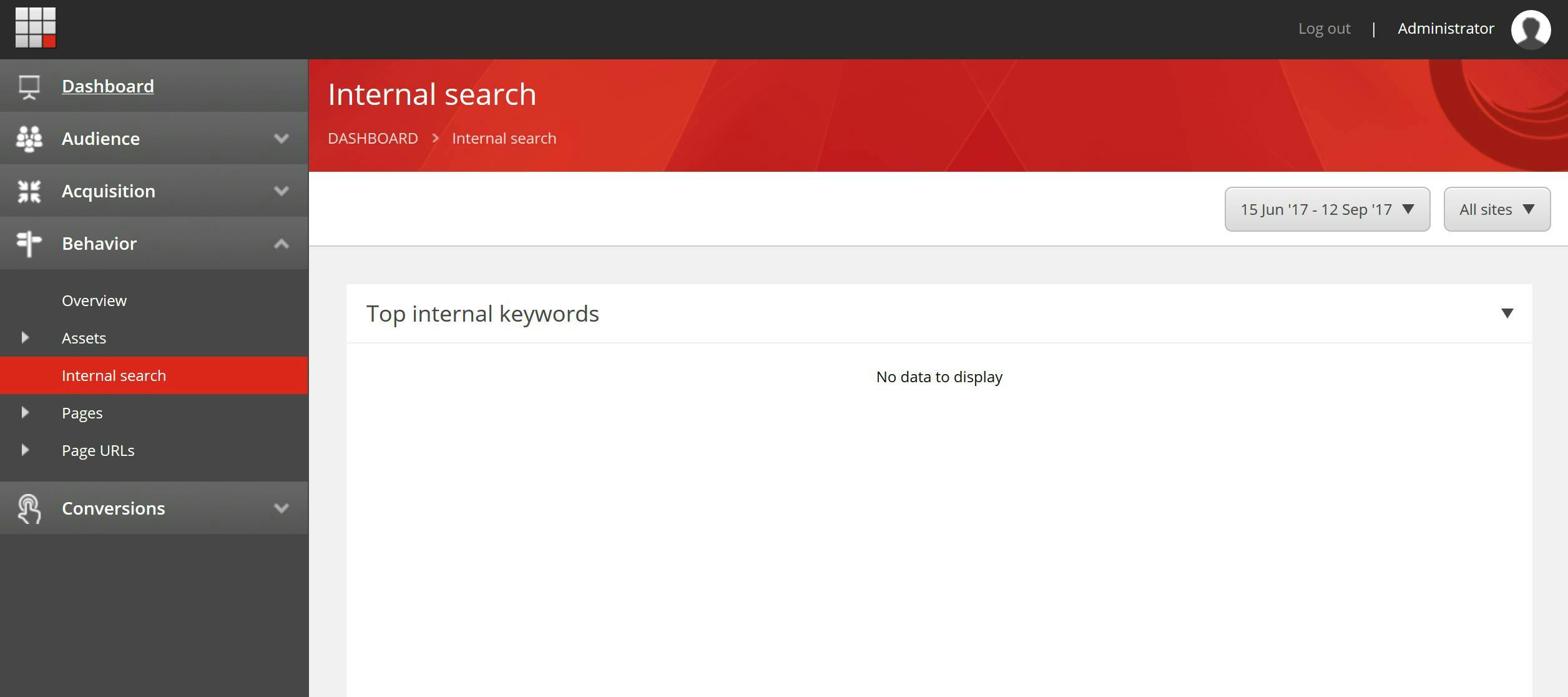
The reason it doesn't show any information is relatively straight forward. Sitecore doesn't know how your site search is going to work and therefore it can't do the data capture part of the process. That part of the process however is actually quite simple to do.
Sitecore has a set of page events that can be registered in the analytics tracker. Some of these like Page Visited will be handled by Sitecore. In this instance the one we are interested in is Search and will we have to register it manually.
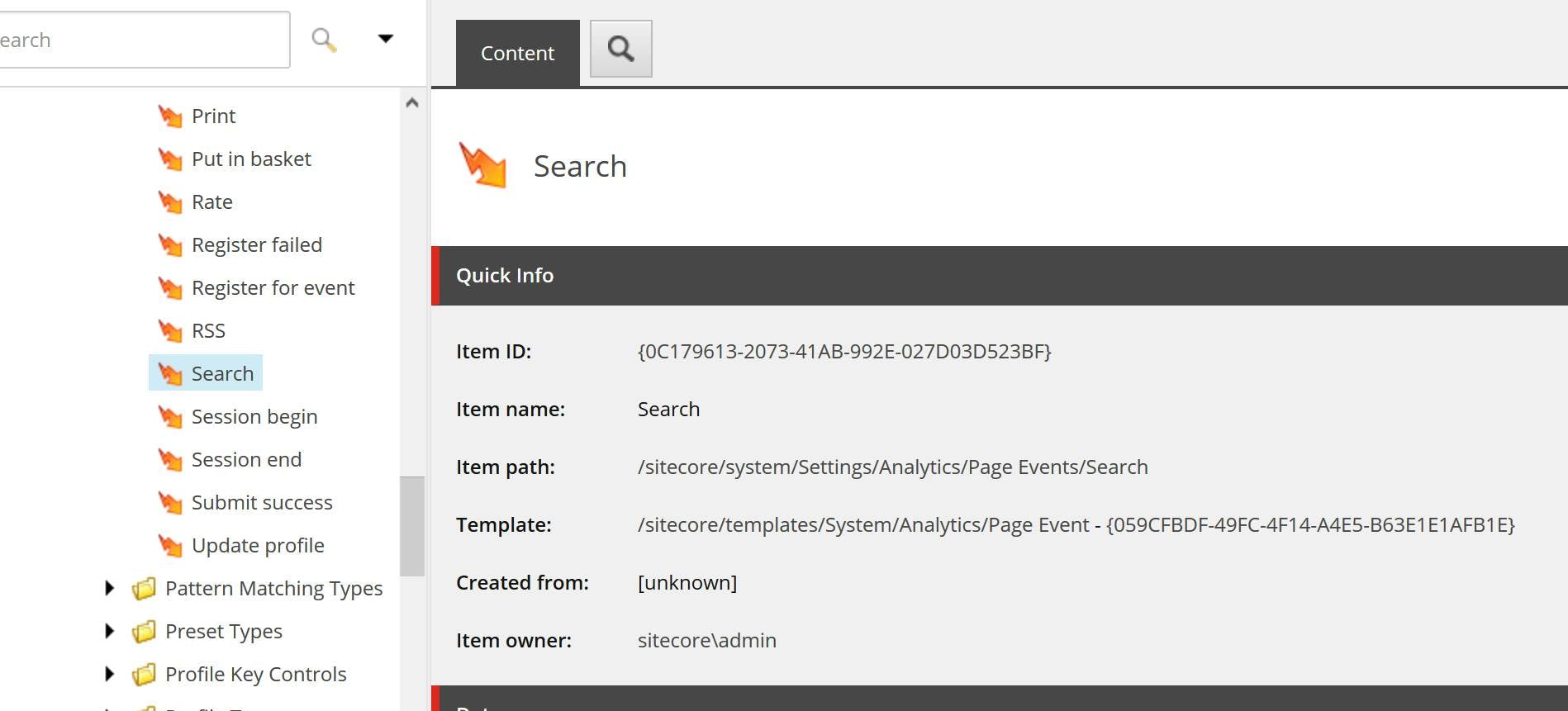
To register the search event use some code like this (note, there is a constant that references the item id of the search event). The query parameter should be populated with the search term the user entered.
1using Sitecore.Analytics;2using Sitecore.Analytics.Data;3using Sitecore.Data.Items;4using Sitecore.Diagnostics;5using SitecoreItemIds;67namespace SitecoreServices8{9 public class SiteSearch10 {11 public static void TrackSiteSearch(Item pageEventItem, string query)12 {13 Assert.ArgumentNotNull(pageEventItem, nameof(pageEventItem));14 Assert.IsNotNull(pageEventItem, $"Cannot find page event: {pageEventItem}");1516 if (Tracker.IsActive)17 {18 var pageEventData = new PageEventData("Search", ContentItemIds.Search)19 {20 ItemId = pageEventItem.ID.ToGuid(),21 Data = query,22 DataKey = query,23 Text = query24 };25 var interaction = Tracker.Current.Session.Interaction;26 if (interaction != null)27 {28 interaction.CurrentPage.Register(pageEventData);29 }30 }31 }32 }33}
Now after triggering the code to be called a few times, your internal search report should start to be populated like this.
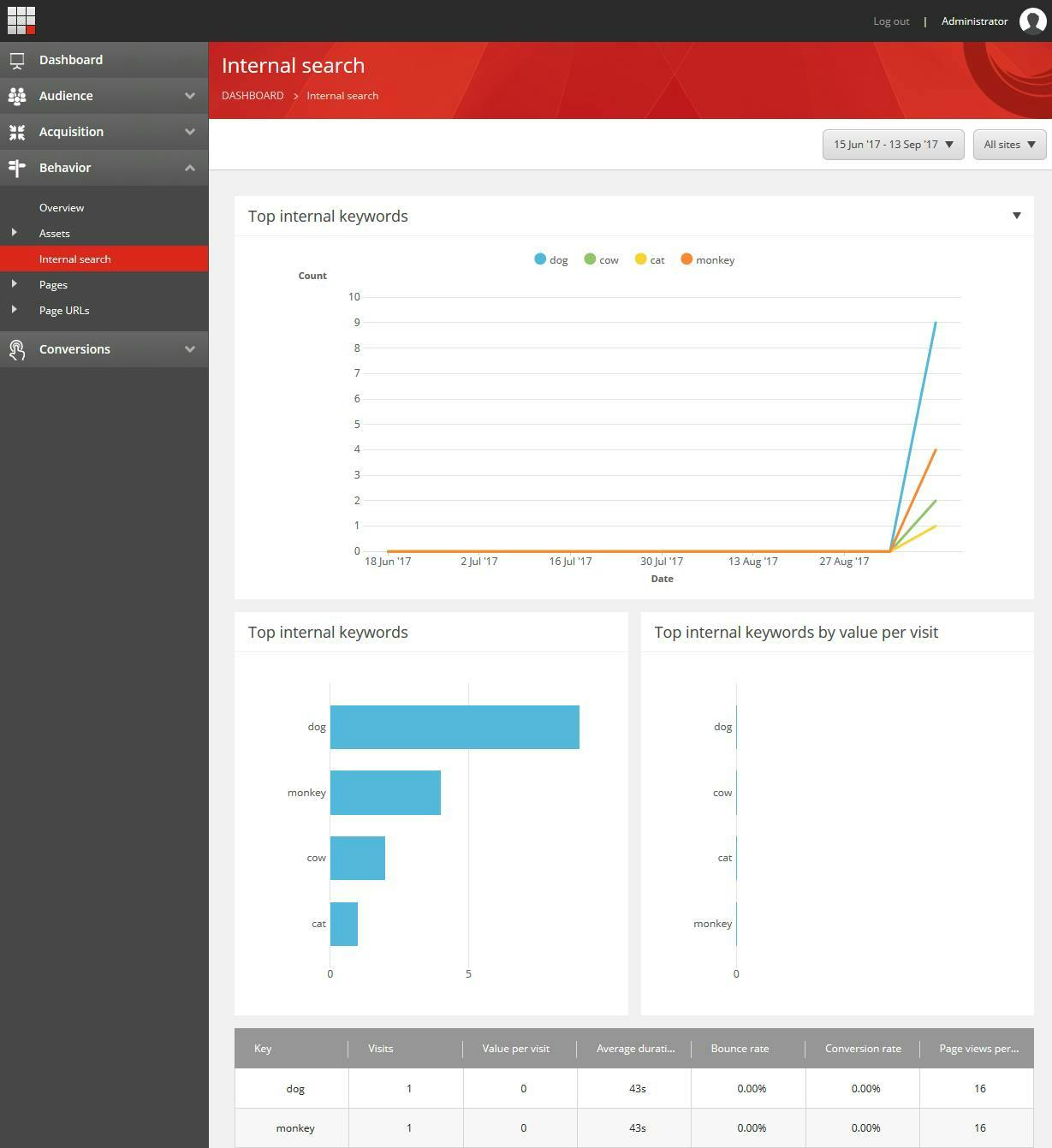