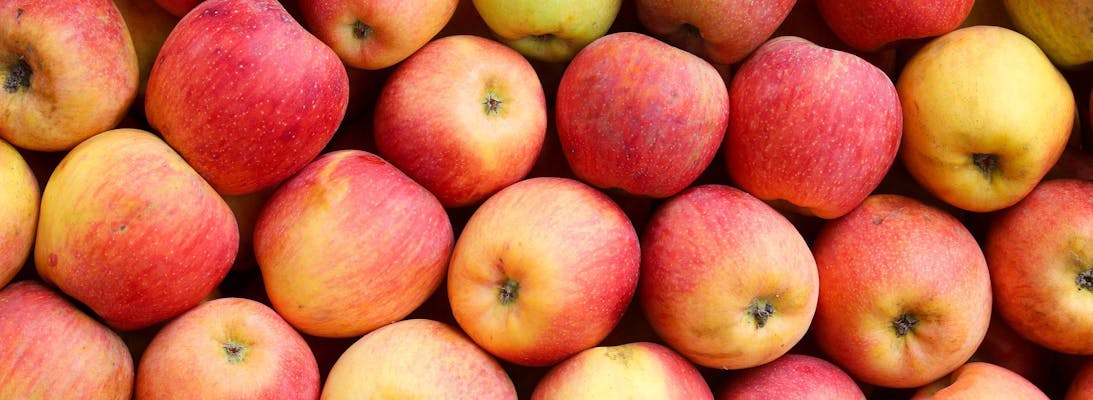
Why should you preload fonts?
There are two ways you can arrive at wanting to preload fonts on a webpage.
The first is your running a lighthouse test for page speed, and scoring badly for cumulative layout shift (CLS). This is the measure of how long elements on the page are shifting position as the page is loaded. This can be impacted by a font load causing elements on a page due to custom fonts having a different size to the default browser font initially used in rendering.
The second is that you can visually see the font switching from default to custom and want to eliminate this.
Now the best way to eliminate both of these issues is to just use browser fonts from the start and that way no font needs to be downloaded in the first place, but the chances are that's not an acceptable solution for your stakeholders.
What is the normal browser behavior?
To use a custom font you first need to define that font as a @font-face in CSS. It will look something like this.
1@font-face {2 font-family: "Bitstream Vera Serif Bold";3 src: url("https://mdn.github.io/css-examples/web-fonts/VeraSeBd.ttf");4}56body {7 font-family: "Bitstream Vera Serif Bold", serif;8}
You will likely define this in a stylesheet file referenced from your HTML page. This means that the browser will first download the HTML page containing text to display, but won't have any way of knowing about the font until the CSS file has been downloaded.
What's worse, the browser is also going to try lazy loading all the font's in. Meaning just because it see's the font-face definition in the stylesheet, it's not going to do anything with it until it knows it's going to be applied to a style on the page, and only then will it start downloading the font.
How to preload a font
To avoid these issues you can tell the browser to preload the font by adding a Link element to the head of the document.
1<head>2 <!-- ... -->3 <link rel="preload" href="/assets/Pacifico-Bold.woff2" as="font" type="font/woff2" crossOrigin='anonymous'>4</head>
This link element tell the browser that the font is going to be used and it should download it as early as possible. Lets break down the attributes:
link - tells the browser to preload the font.
href - the url of the file to download.
as and type- what type of file this is. In this case its a font with a mime type of font/woff2.
crossOrigin - an interesting quirk with browsers, unless you set this the file wont get downloaded even if your hosting the font yourself.
Best Practices with Fonts
Here are some more best practices to follow with fonts.
Minimize the number of custom fonts
The best way to avoid issues with loading font's is to use as few custom fonts as possible. Essentially the more you add the more that will need to be downloaded.
Although fonts can be preloaded via preloading, you are only ever optimizing the critical path to show the webpage, it won't do anything to reduce the total data needing to be downloaded. A browser is also limited in the number of requests it will make at a time, meaning the more files there are, the more that will ultimately get queued.
Only Preload the main font
To ensure browser support your font-face definition might actually contain multiple font files of different types.
1@font-face {2 font-family: 'MyWebFont';3 src: url('myfont.woff2') format('woff2'),4 url('myfont.woff') format('woff');5}
When the browser reads these definitions they will pick the first in the list with a format that they support and ignore the rest. However if you add a Link tag to preload each of them, the browser will have no way of knowing it is an order of preference and download them all. Therefore you should only preload the first in the list. This will likely be a woff2 that's supported by basically all modern browsers.