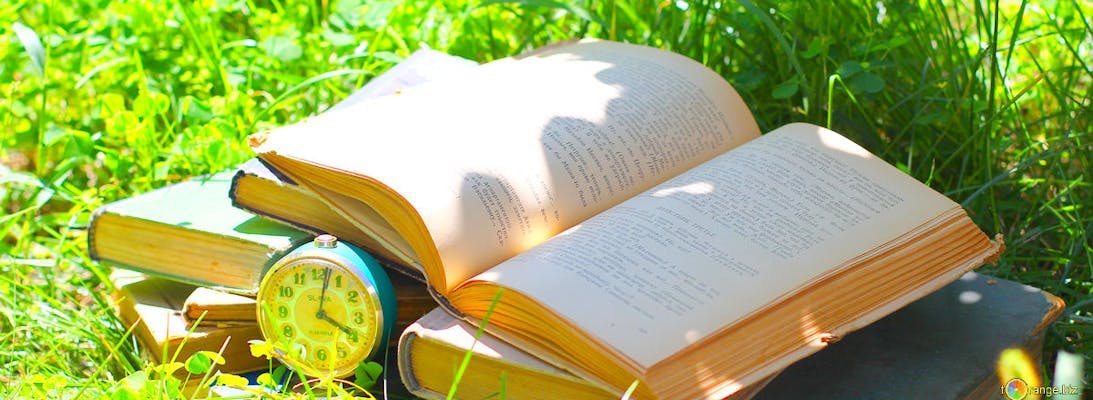
Invert list selection with Sitecore PowerShell
I recently needed to run a script on a block of Sitecore content in invert the selection of a checklist and multilist. As I couldn't find any example of how to do this at the time, I thought I'd share what I wrote.
1#script to update tier2Get-ChildItem -r -Path "master:\content\Home" -Language * | ForEach-Object {3 if ($_.PSobject.Properties.name -match "Tier") {4 [String[]]$tiers = $_.Tier -split "\|"56 $_.Editing.BeginEdit()7 $newtiers = Get-ChildItem 'master:\content\Lookups\Tiers\' | Where-Object { $tiers -notcontains $_.Id }8 $_.Tier = $newtiers.Id -join "|"9 $_.Editing.EndEdit()10 }11}
Get-ChildItem -r -Path "master:\content\Home" -Language * | ForEach-Object {
This line is getting the child items from the home node in the master db. The -r specified that it should be recursive and the -Language * specifies to include all languages. The results are then piped to a for each loop.
if ($_.PSobject.Properties.name -match "Tier") {
The field I needed to update was called Tier, as this was included in multiple templates I checked that the object included a field called Tier, rather than checking the template types.
[String[]]$tiers = $_.Tier -split "\|"
List fields in Sitecore get stored as a pipe separated list of the item Id's for the selected items. In order to do a comparison in Powershell I needed to turn the string back into an array using the split command. Notice the backslash that is needed before the pipe.
$_.Editing.BeginEdit()
To edit an item you need to begin an edit
$newtiers = Get-ChildItem 'master:\content\Components\Lookups\Tiers\' | Where-Object { $tiers -notcontains $_.Id }
This is where we get the new list of tiers to set as the selected ones for the item. The Get-ChildItem command is retrieving the original options that could be selected and the where-object statement is then excluding the ones that are in the $tiers array we just made.
$_.Tier = $newtiers.Id -join "|"
To save the new list we need to convert the results of the query into a pipe separated list using a join.
$_.Editing.EndEdit() } }
End the editing to save and close the the if and loop statements.