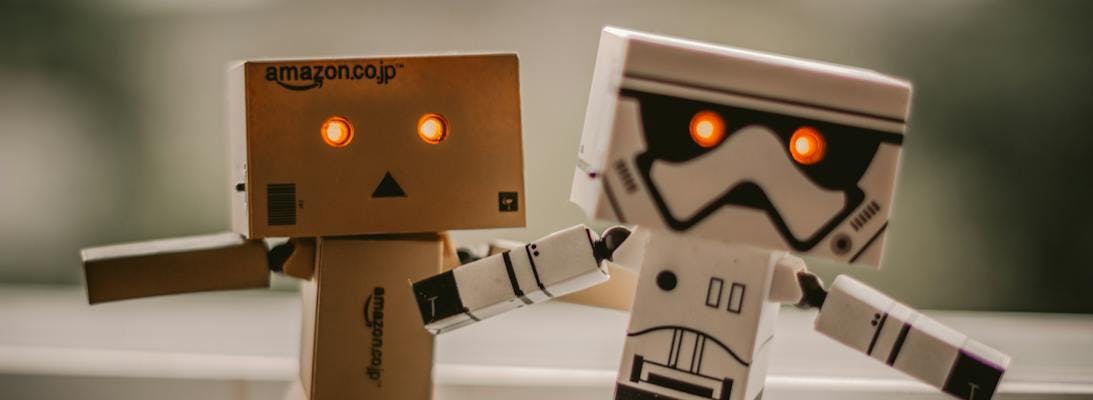
API Routes stopped working in Sitecore 9
We recently undertook a Sitecore 9 upgrade and one issue we encountered was controllers we had set up for API routes stopped working on content delivery servers. Content management and single server setups were unaffected.
Our routes had been set up by the controllers inheriting from SitecoreController and then using the default route which Sitecore would create. e.g. /api/sitecore/foo/getFoo
1public class FooController : SitecoreController2{3 ...4 [System.Web.Http.HttpPost]5 public ActionResult GetFoo(FooModel queryModel)6 {7 ...8 }9}
MVC.LegalRoutes
After a bit of investigation we found a new setting in Sitecore 9 called MVC.LegalRoutes. From the documentation:
MVC: Pipe separated list of route URL's that are not valid for use with Sitecore.Mvc.
For instance, the default ASP.NET route ({controller}/{action}/{id}) catches most requests that are actually meant to be handled by the default Sitecore route.
Default: "{controller}/{action}/{id}"
If we added our route to the list everything started to work ok.
1<setting name="Mvc.LegalRoutes" value="|Sitecore.Mvc:sitecore/shell/api/sitecore/{controller}/{action}|Sitecore.Mvc.Api:/api/sitecore/{controller}/{action}|" />
A different approach
Not wanting to just change random settings we stumble across we contacted Sitecore support to see what they thought.
The route 'api/sitecore/{controller}/{action}' is a pre-defined Sitecore SPEAK route for Sitecore Client usage. So when trying to access on a content delivery server where the Sitecore Client is disabled, it no longer works.
So to get around the issue we can start registering the route on content delivery servers through the Global.asax file.
1public override void Application_Start(object sender, EventArgs args)2{3 base.Application_Start(sender, args);45 if (System.Configuration.ConfigurationManager.AppSettings["role:define"] == "ContentDelivery")6 {7 System.Web.Routing.RouteTable.Routes.MapRoute("Sitecore.Speak.Commands", "api/sitecore/{controller}/{action}");8 }9}