Item and Field Names in Sitecore
Item and Field names in Sitecore might sound like a simple subject, but there are a few options that you may not be aware of.
Item Names
When you create an item in Sitecore it will ask you for a name to give the item. That name then appears in the content tree and at the top of the details pane to identify what your looking at.
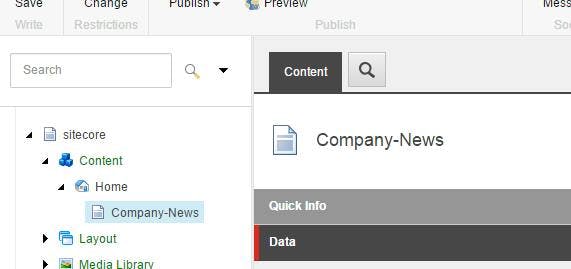
It's also used for the URL in the front end of your site.
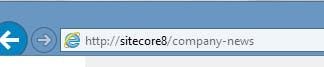
All fairly basic stuff. However in this example you will see that I've placed a hyphen between "Company" and "News" to make the the URL a little more friendly, rather than having a space or no gap at all. While good for the URL it comes at a sacrifice to the admin experience where a gap would be nicer.
On a multilingual site the URL's are also still using this same name. If your languages are English and US English that may be ok, but if your second language is French then they would probably prefer a URL in French.
Display Names
This is where Display Names come in. By setting a Display Name for your item you can configure a name to show in the admin that contains the space which can also differ per language.
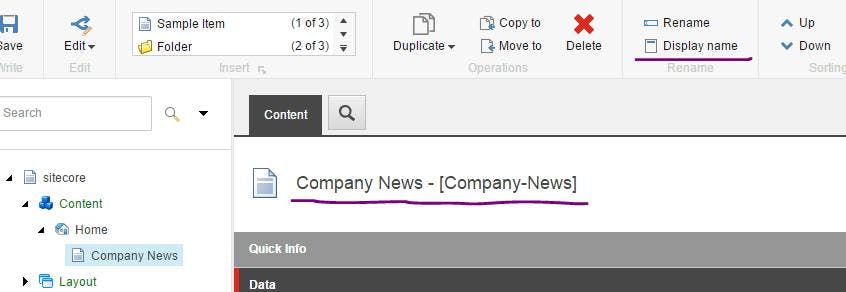
However at this point the URL on the front end of your site will still be "Company News". Through a config setting though you can set Sitecore to use item display names rather than the item name when constructing a URL.
1<configuration xmlns:patch="http://www.sitecore.net/xmlconfig/">2 <sitecore>3 <linkManager>4 <providers>5 <add name="sitecore">6 <patch:attribute name="useDisplayName">true</patch:attribute>7 </add>8 </providers>9 </linkManager>10 </sitecore>11</configuration>
Of course at this point your URL will be back to having a space within it.
Field Names
When you define a template in Sitecore you create add a section name and then add a field within that section. All simple stuff, however what you generally find is you have to prefix the name of your fields with the name of the section. This is because when it comes to accessing the field data in code, the sections don't exist and you need a way to avoid having two fields just called "Title" or "Text".
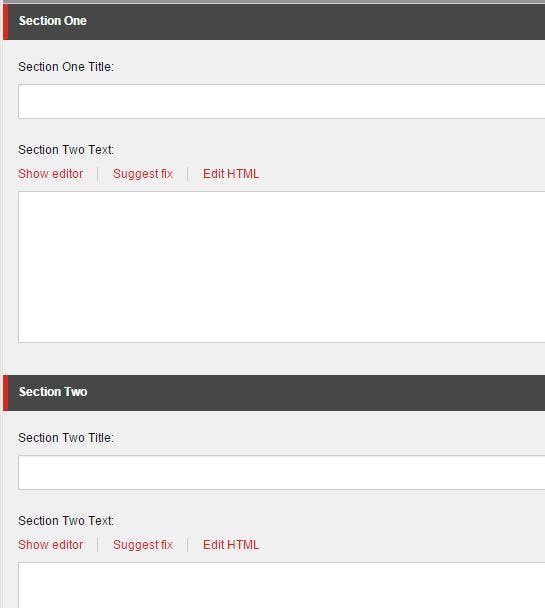
While this solves the issue from a code perspective, for content editors it's far from ideal.
Fortunately there is a solution to this. By navigating to the actual field item within the content tree, we can specify a Title for the field that will be used in the editor rather than its name.
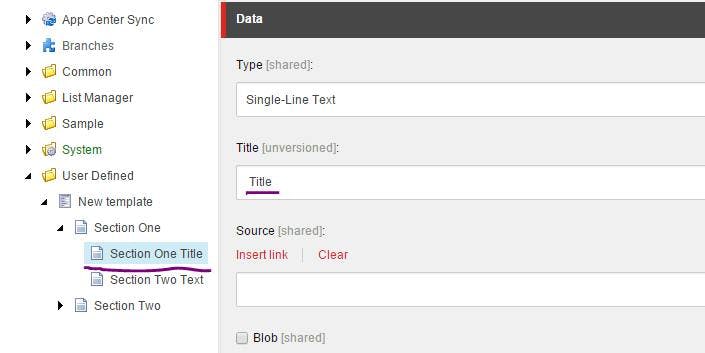
On top of that if you click the Configure tab and select Help, you can enter some help text that will appear next to the field name in the content editor.
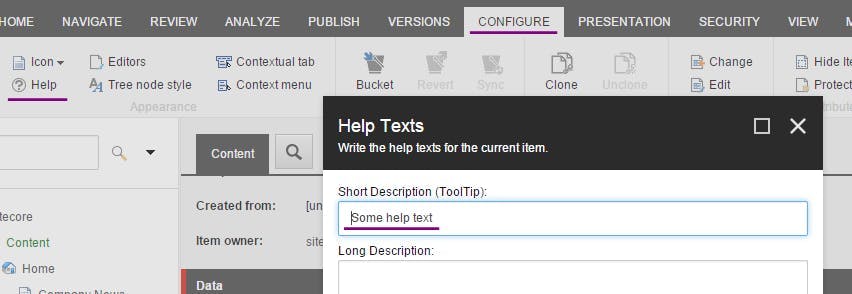
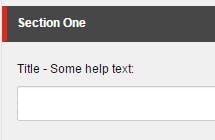